What's "new" in JS
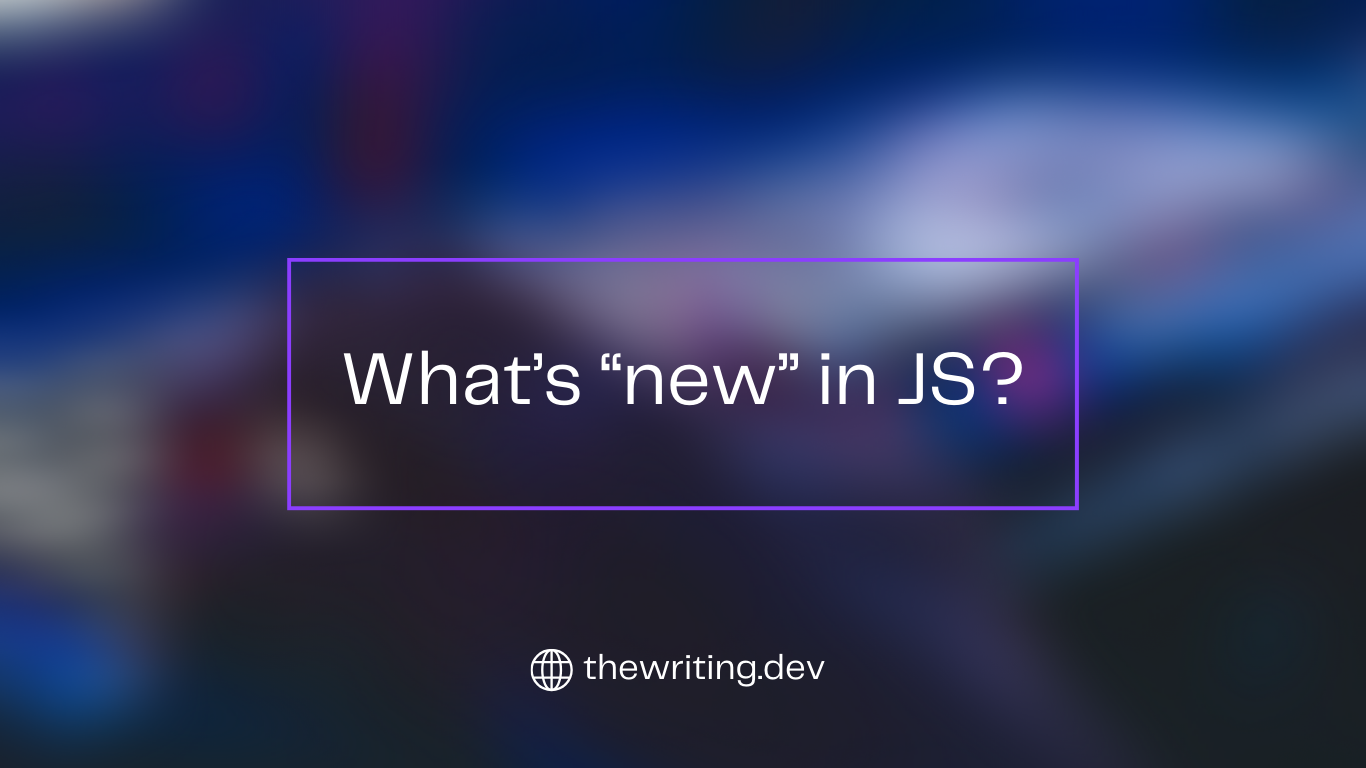
Everyone knows that JS is a multi-paradigm language. However, did you know to what extent? JS allows you to encapsulate your state, create abstractions and perform using both - Functions and Objects. How, you may ask? Let's find out.
The new
keyword lets developers create an instance of a class or a Javascript Object that has a constructor function.
function Car(make, model, year) {
this.make = make;
this.model = model;
this.year = year;
}
const car1 = new Car('Eagle', 'Talon TSi', 1993);
console.log(car1.make);
// Expected output: "Eagle"
Here's how it works with classes and objects:
Class
This is the well-known way of using "new". It simply invokes the constructor and returns a new instance of the class as an object.
class Person {
constructor(name) {
this.name = name;
}
greet() {
console.log(`Hello, my name is ${this.name}`);
}
}
const p = new Person("Caroline");
p.greet(); // Hello, my name is Caroline
Function
The "new" keyword is not just limited to classes, but was originally used with functions. When applied to a function, it does different things depending on what's returned.
- Non-primitive Types - If the function returns a non-primitive, the return value becomes the result of the whole
new
expression.
function Foo(bar1, bar2) {
this.bar1 = bar1;
this.bar2 = bar2;
return {
bar1,
bar2
};
}
// myFoo is just the returned object without access to this values
const myFoo = new Foo(1,2);
- Primitive Types - If the function returns a primitive, the return value is an instance of the function, similar to how
class
works.
function Car() {}
const car1 = new Car();
console.log(car1.color); // undefined
Car.prototype.color = "original color";
console.log(car1.color); // 'original color'
This way, you can use the new
keyword to follow Object Oriented Principles using functions or classes. Try it out yourselves, or read the documentation in more detail on MDN.