Building Your First MCP Server
Build your first MCP server and extend AI assistants with your own tools. This beginner's guide shows how to connect external services to Claude and other LLMs, unlocking powerful capabilities.
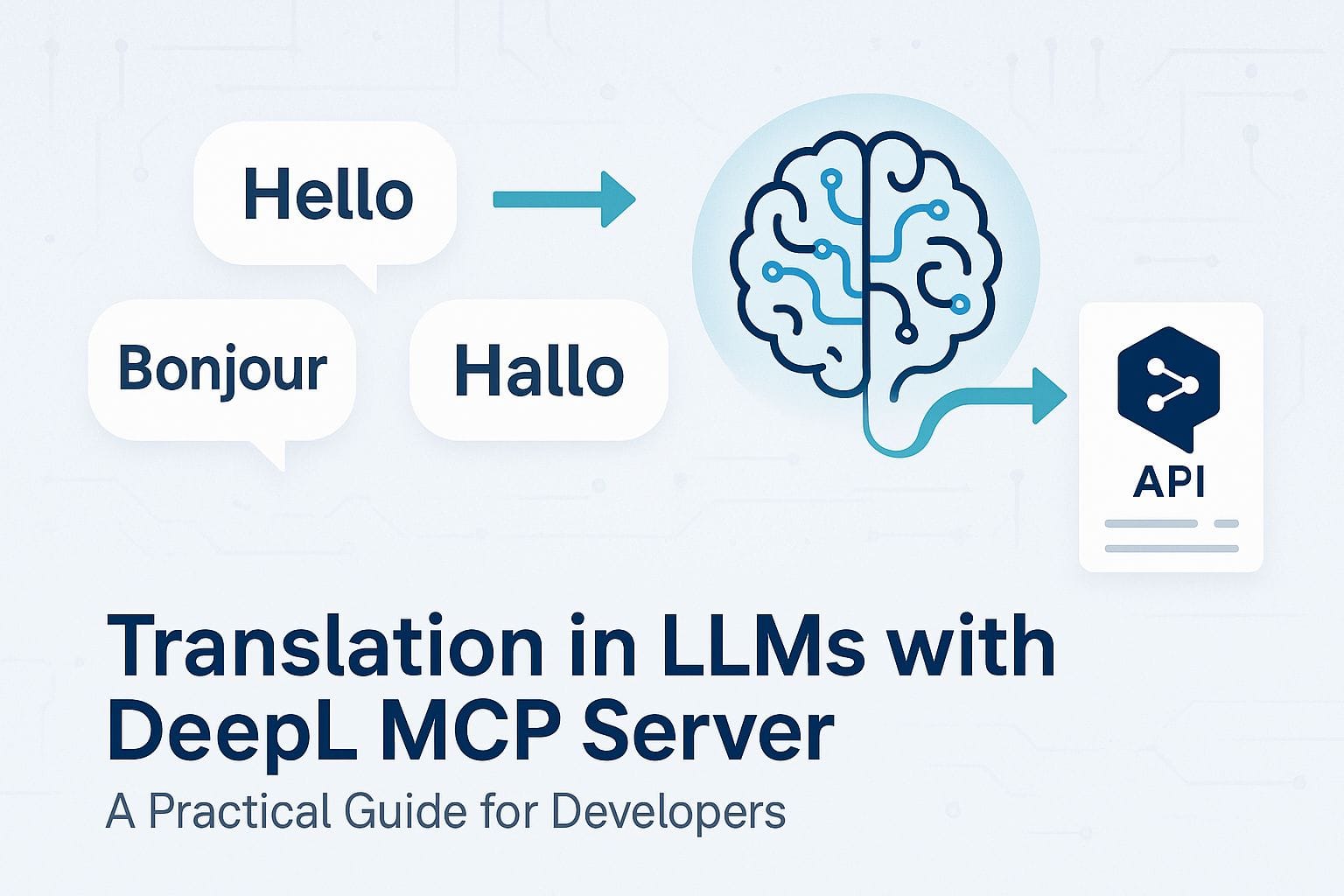
Ever wondered how to give AI assistants like Claude or GitHub Copilot access to your own code or tools? The Model Context Protocol (MCP) makes this possible, and I've been experimenting with it to create powerful AI integrations.
In this guide, I'll walk you through creating your first MCP server - a bridge that connects specialized services with large language models. By the end, you'll understand how to extend AI capabilities beyond their out-of-the-box functionality.
What is MCP and Why It Matters
Think of MCP as a universal connector for AI tools - similar to how USB standardized hardware connections. Before MCP, developers needed custom integrations for each AI platform they wanted to connect with external services. Now, we have a standardized way for AI assistants to discover and use external tools.
This protocol, introduced by Anthropic, allows Claude and other compatible LLM agents to seamlessly access specialized capabilities from external services through a consistent interface.
Getting Started with Your MCP Server
The Setup
First, let's create a new Node.js project and install the necessary packages:
mkdir mcp-server-project
cd mcp-server-project
npm init -y
npm install @modelcontextprotocol/sdk zod
We're using two key libraries:
- The MCP SDK handles the communication protocol
- Zod provides schema validation for our tool parameters
Creating the Server
Now, let's build a simple MCP server. Create a file named server.mjs
:
import { McpServer, StdioServerTransport } from '@modelcontextprotocol/sdk';
import { z } from 'zod';
// Define a basic tool
const myTools = [
{
name: 'hello-world',
description: 'A simple greeting tool',
parameters: z.object({
name: z.string().describe('The name to greet'),
}),
handler: async ({ name }) => {
return `Hello, ${name}! Welcome to the world of MCP.`;
},
},
];
// Initialize and start the server
const server = new McpServer({
tools: myTools,
});
const transport = new StdioServerTransport();
server.listen(transport);
console.error('MCP Server is now running...');
This creates a minimal MCP server with a single tool that generates a greeting message. The real power comes when you replace this with calls to external APIs or services.
Integrating with Claude Desktop
To use your new MCP server with Claude Desktop:
- Locate your Claude configuration file:
- On Mac:
~/Library/Application Support/Claude/claude_desktop_config.json
- On Windows:
%AppData%\Claude\claude_desktop_config.json
- On Linux:
~/.config/Claude/claude_desktop_config.json
- On Mac:
- Configure your server:
{
"servers": [
{
"name": "My MCP Server",
"command": ["node", "/full/path/to/your/server.mjs"],
"env": {
"NODE_ENV": "production"
}
}
]
}
- Restart Claude Desktop
When you open Claude, your tool should appear in the tools menu. You can now ask Claude to use your custom tool!
Let's break down what makes an MCP server work.
The Tools Array
Each tool needs four elements:
- A unique name and clear description to help the LLM understand what the tool does.
- A parameter schema (using Zod) for input handling
- A handler function that does the actual work
The Transport Layer
The StdioServerTransport
handles communication between your server and MCP clients like Claude. For production use, you might want to implement more robust transport options.
Error Handling
While not shown in our simple example, proper error handling is crucial for production servers. Your handlers should gracefully manage API failures and provide helpful error messages for the LLM.
This is just the beginning of what you can build with MCP. For a more comprehensive example that shows how to integrate translation capabilities using the DeepL API, check out my detailed guide on the DeepL Developers site:
The complete guide explores advanced topics like handling API authentication, managing rate limits, and structuring a production-ready MCP server.
What will you build with MCP?